#include <project.h>

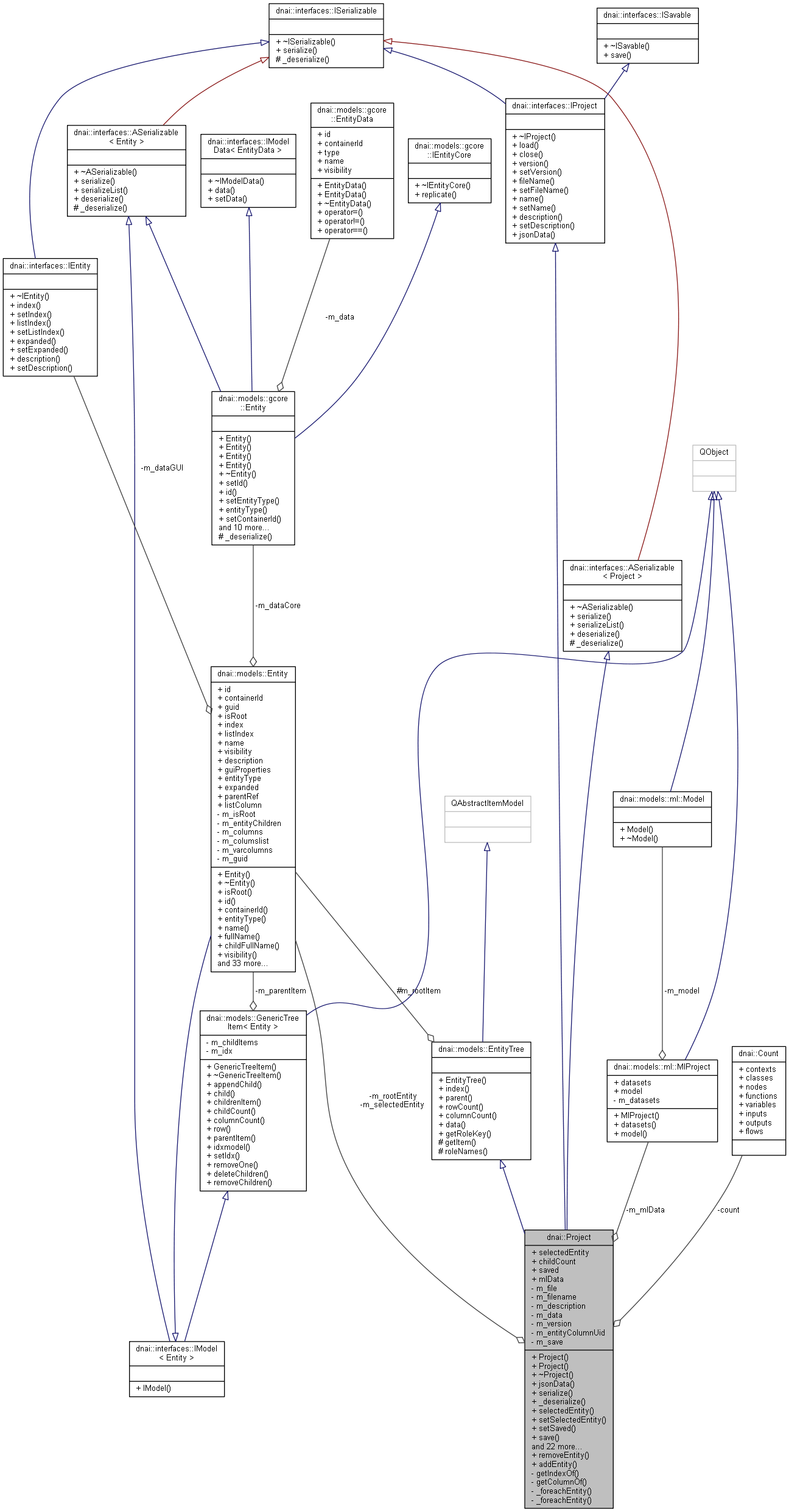
Public Slots | |
void | removeEntity (dnai::models::Entity *entity) |
void | addEntity (dnai::models::Entity *entity) |
Signals | |
void | selectedEntityChanged (models::Entity *entity) |
void | entityAdded (models::Entity *entity) |
void | entityRemoved (models::Entity *entity) |
void | savedChanged (bool saveState) |
Public Member Functions | |
Project () | |
Project (const QString &filename) | |
~Project () | |
const QJsonObject & | jsonData () const override |
void | serialize (QJsonObject &obj) const override |
Implement this function to serialize into QJsonObject. More... | |
void | _deserialize (const QJsonObject &obj) override |
Implement this function in order to use deserialize(const QJsonObject &obj) More... | |
models::Entity * | selectedEntity () const |
void | setSelectedEntity (models::Entity *entity) |
void | setSaved (bool) |
Q_INVOKABLE void | save () override |
bool | saved () const |
void | load (const QString &path) override |
void | loadFromJson (const QJsonObject &obj) |
void | close () override |
const QString & | version () const override |
void | setVersion (const QString &version) override |
const QString & | name () const override |
void | setName (const QString &name) override |
const QString & | description () const override |
void | setDescription (const QString &name) override |
const QString & | fileName () const override |
void | setFileName (const QString &name) override |
void | foreachEntity (const std::function< void(models::Entity *)> &func) const |
int | childCount () const |
models::Entity & | getRoot () const |
models::ml::MlProject * | mlData () |
models::ml::MlProject & | MlData () |
Q_INVOKABLE int | expandedRows (const QModelIndex &parent) const |
Project::expandedRows Count the number of children to display in treeview. More... | |
Q_INVOKABLE int | expandedRows () const |
Project::expandedRows Count the expanded rows of the entire project in the tree view. More... | |
Q_INVOKABLE void | addEntityColumnUid (quint32 parentId, QString const &name, QString const &listIndex) |
Q_INVOKABLE QString | generateUniqueChildName (dnai::models::Entity *parent) const |
template<> | |
void | _foreachEntity (models::Entity *root, const std::function< void(models::Entity *)> &func) const |
![]() | |
EntityTree (QObject *parent=nullptr) | |
QModelIndex | index (int row, int column, const QModelIndex &parent) const override |
QModelIndex | parent (const QModelIndex &child) const override |
int | rowCount (const QModelIndex &parent) const override |
int | columnCount (const QModelIndex &parent) const override |
QVariant | data (const QModelIndex &index, int role) const override |
Q_INVOKABLE int | getRoleKey (QString rolename) const |
![]() | |
virtual | ~ASerializable ()=default |
QJsonArray | serializeList (const QList< DataType * > &datalist) const |
![]() | |
virtual | ~IProject ()=default |
![]() | |
virtual | ~ISavable ()=default |
Properties | |
dnai::models::Entity | selectedEntity |
int | childCount |
bool | saved |
dnai::models::ml::MlProject | mlData |
Private Member Functions | |
QModelIndex | getIndexOf (models::Entity *e) const |
models::Column * | getColumnOf (models::Entity *e) |
template<class T > | |
T | _foreachEntity (models::Entity *root, const std::function< T(models::Entity *)> &func) const |
template<> | |
int | _foreachEntity (models::Entity *root, const std::function< int(models::Entity *)> &func) const |
Private Attributes | |
QFile * | m_file |
Count | count |
models::Entity * | m_selectedEntity {} |
QString | m_filename |
QString | m_description |
QJsonObject | m_data |
QString | m_version |
models::Entity * | m_rootEntity |
QMap< QString, QString > | m_entityColumnUid |
models::ml::MlProject | m_mlData |
bool | m_save = true |
Additional Inherited Members | |
![]() | |
enum | ROLES { ID = Qt::UserRole + 1, TYPE, CONTAINER_ID, NAME, VISIBILITY, INDEX, LISTINDEX, DESCRIPTION, CORE_MODEL, GUI_MODEL, MODEL, EXPANDED, LIST_COLUMN } |
![]() | |
static Project * | deserialize (const QJsonObject &obj, Args &...args) |
This function deserialize into a new instance of type T *. More... | |
![]() | |
Entity * | getItem (const QModelIndex &index) const |
virtual QHash< int, QByteArray > | roleNames () const override |
![]() | |
Entity * | m_rootItem |
Constructor & Destructor Documentation
dnai::Project::Project | ( | ) |
dnai::Project::Project | ( | const QString & | filename | ) |
dnai::Project::~Project | ( | ) |
Member Function Documentation
|
overridevirtual |
Implement this function in order to use deserialize(const QJsonObject &obj)
- Parameters
-
obj
Implements dnai::interfaces::ASerializable< Project >.
|
private |
|
inline |
|
private |
|
slot |
void dnai::Project::addEntityColumnUid | ( | quint32 | parentId, |
QString const & | name, | ||
QString const & | listIndex | ||
) |
int dnai::Project::childCount | ( | ) | const |
|
overridevirtual |
Implements dnai::interfaces::IProject.
|
overridevirtual |
Implements dnai::interfaces::IProject.
|
signal |
|
signal |
int dnai::Project::expandedRows | ( | const QModelIndex & | parent | ) | const |
Project::expandedRows Count the number of children to display in treeview.
It do a breadth-first order traversal from the given node in each expanded children but stops depth research at first children collapsed
- Parameters
-
parent The item that is expanded/collapse
- Returns
- Number of child to display
int dnai::Project::expandedRows | ( | ) | const |
Project::expandedRows Count the expanded rows of the entire project in the tree view.
- Returns
- Count of expanded rows from root
|
overridevirtual |
Implements dnai::interfaces::IProject.
void dnai::Project::foreachEntity | ( | const std::function< void(models::Entity *)> & | func | ) | const |
QString dnai::Project::generateUniqueChildName | ( | dnai::models::Entity * | parent | ) | const |
|
private |
|
private |
models::Entity & dnai::Project::getRoot | ( | ) | const |
|
overridevirtual |
Implements dnai::interfaces::IProject.
|
overridevirtual |
Implements dnai::interfaces::IProject.
void dnai::Project::loadFromJson | ( | const QJsonObject & | obj | ) |
models::ml::MlProject* dnai::Project::mlData | ( | ) |
models::ml::MlProject & dnai::Project::MlData | ( | ) |
|
overridevirtual |
Implements dnai::interfaces::IProject.
|
slot |
|
overridevirtual |
Implements dnai::interfaces::ISavable.
bool dnai::Project::saved | ( | ) | const |
|
signal |
models::Entity* dnai::Project::selectedEntity | ( | ) | const |
|
signal |
|
overridevirtual |
Implement this function to serialize into QJsonObject.
- Parameters
-
obj
Implements dnai::interfaces::ASerializable< Project >.
|
overridevirtual |
Implements dnai::interfaces::IProject.
|
overridevirtual |
Implements dnai::interfaces::IProject.
|
overridevirtual |
Implements dnai::interfaces::IProject.
void dnai::Project::setSaved | ( | bool | save | ) |
void dnai::Project::setSelectedEntity | ( | models::Entity * | entity | ) |
|
overridevirtual |
Implements dnai::interfaces::IProject.
|
overridevirtual |
Implements dnai::interfaces::IProject.
Member Data Documentation
|
private |
|
private |
|
private |
|
private |
|
private |
|
private |
|
private |
|
private |
|
private |
|
private |
|
private |
Property Documentation
|
read |
|
read |
|
readwrite |
|
readwrite |
The documentation for this class was generated from the following files:
- Gui/app/include/dnai/project.h
- Gui/app/GeneratedFiles/Debug/moc_project.cpp
- Gui/app/src/dnai/project.cpp