Public Member Functions |
Protected Member Functions |
Properties |
Private Attributes |
List of all members
dnai::models::gui::Instruction Class Reference
#include <instruction.h>
Inheritance diagram for dnai::models::gui::Instruction:
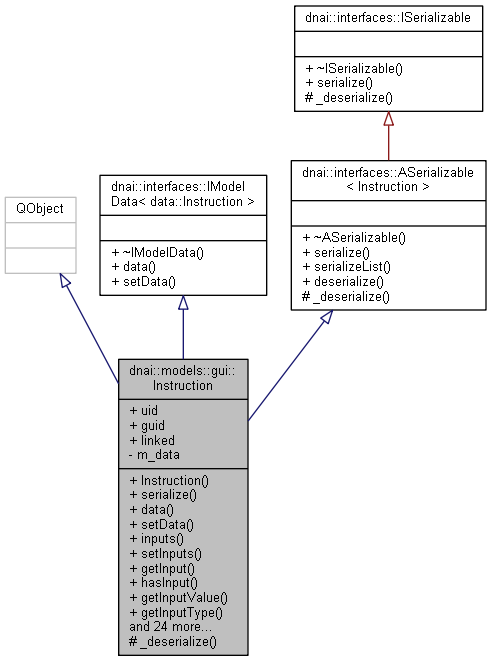
Collaboration diagram for dnai::models::gui::Instruction:
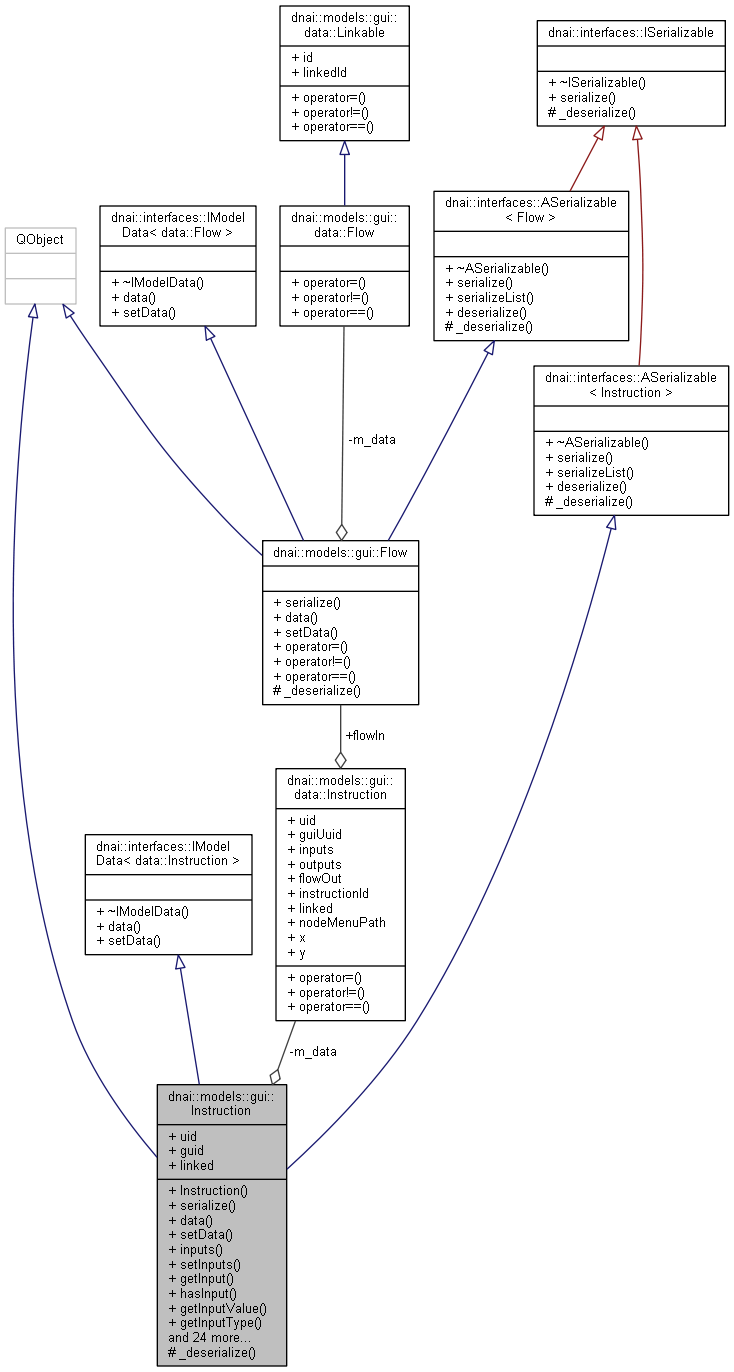
Public Member Functions | |
Instruction (QObject *parent=nullptr) | |
virtual void | serialize (QJsonObject &obj) const override |
Implement this function to serialize into QJsonObject. More... | |
const data::Instruction & | data () const override |
Return the data component. More... | |
bool | setData (const data::Instruction &data) override |
Q_INVOKABLE const QList< models::gui::Input * > & | inputs () const |
bool | setInputs (const QList< models::gui::Input * > &inputs) |
Q_INVOKABLE models::gui::Input * | getInput (QString const &name) const |
bool | hasInput (QString const &name) const |
Q_INVOKABLE QString | getInputValue (QString const &name) const |
Q_INVOKABLE QString | getInputType (QString const &name) const |
const QList< models::gui::Output * > & | outputs () const |
bool | hasOutput (QString const &name) const |
bool | setOutputs (const QList< models::gui::Output * > &outputs) |
Q_INVOKABLE models::gui::Output * | getOutput (QString const &name) const |
Q_INVOKABLE QString | getOutputType (QString const &name) const |
models::gui::Flow * | flowIn () const |
bool | setFlowIn (models::gui::Flow *flow) |
const QList< models::gui::Flow * > & | flowOut () const |
bool | setFlowOut (const QList< models::gui::Flow * > &flow) |
qint32 | instruction_id () const |
bool | setInstructionId (qint32 id) |
quint32 | Uid () const |
bool | setUid (quint32 id) |
QList< QString > const & | linked () const |
bool | setLinkedEntities (QList< QString > const &value) |
const QUuid & | guiUuid () const |
QString | guid () const |
bool | setGuiUuid (const QUuid &value) |
const QString & | nodeMenuPath () const |
bool | setNodeMenuPath (QString const &value) |
qint32 | x () const |
Q_INVOKABLE bool | setX (qint32 x) |
qint32 | y () const |
Q_INVOKABLE bool | setY (qint32 y) |
![]() | |
virtual | ~IModelData ()=default |
virtual bool | setData (const data::Instruction &data)=0 |
set the data component of this object More... | |
![]() | |
virtual | ~ASerializable ()=default |
QJsonArray | serializeList (const QList< DataType * > &datalist) const |
Protected Member Functions | |
virtual void | _deserialize (const QJsonObject &obj) override |
Implement this function in order to use deserialize(const QJsonObject &obj) More... | |
Properties | |
qint32 | uid |
QString | guid |
QList< QString > | linked |
Private Attributes | |
data::Instruction | m_data |
Additional Inherited Members | |
![]() | |
static Instruction * | deserialize (const QJsonObject &obj, Args &...args) |
This function deserialize into a new instance of type T *. More... | |
Constructor & Destructor Documentation
|
explicit |
Member Function Documentation
|
overrideprotectedvirtual |
Implement this function in order to use deserialize(const QJsonObject &obj)
- Parameters
-
obj
Implements dnai::interfaces::ASerializable< Instruction >.
|
overridevirtual |
Return the data component.
- Returns
- const T& data
Implements dnai::interfaces::IModelData< data::Instruction >.
models::gui::Flow * dnai::models::gui::Instruction::flowIn | ( | ) | const |
const QList< models::gui::Flow * > & dnai::models::gui::Instruction::flowOut | ( | ) | const |
Input * dnai::models::gui::Instruction::getInput | ( | QString const & | name | ) | const |
QString dnai::models::gui::Instruction::getInputType | ( | QString const & | name | ) | const |
QString dnai::models::gui::Instruction::getInputValue | ( | QString const & | name | ) | const |
Output * dnai::models::gui::Instruction::getOutput | ( | QString const & | name | ) | const |
QString dnai::models::gui::Instruction::getOutputType | ( | QString const & | name | ) | const |
QString dnai::models::gui::Instruction::guid | ( | ) | const |
const QUuid & dnai::models::gui::Instruction::guiUuid | ( | ) | const |
bool dnai::models::gui::Instruction::hasInput | ( | QString const & | name | ) | const |
bool dnai::models::gui::Instruction::hasOutput | ( | QString const & | name | ) | const |
const QList< models::gui::Input * > & dnai::models::gui::Instruction::inputs | ( | ) | const |
qint32 dnai::models::gui::Instruction::instruction_id | ( | ) | const |
QList<QString> const& dnai::models::gui::Instruction::linked | ( | ) | const |
const QString & dnai::models::gui::Instruction::nodeMenuPath | ( | ) | const |
const QList< models::gui::Output * > & dnai::models::gui::Instruction::outputs | ( | ) | const |
|
overridevirtual |
Implement this function to serialize into QJsonObject.
- Parameters
-
obj
Implements dnai::interfaces::ASerializable< Instruction >.
|
override |
bool dnai::models::gui::Instruction::setFlowIn | ( | models::gui::Flow * | flow | ) |
bool dnai::models::gui::Instruction::setFlowOut | ( | const QList< models::gui::Flow * > & | flow | ) |
bool dnai::models::gui::Instruction::setGuiUuid | ( | const QUuid & | value | ) |
bool dnai::models::gui::Instruction::setInputs | ( | const QList< models::gui::Input * > & | inputs | ) |
bool dnai::models::gui::Instruction::setInstructionId | ( | qint32 | id | ) |
bool dnai::models::gui::Instruction::setLinkedEntities | ( | QList< QString > const & | value | ) |
bool dnai::models::gui::Instruction::setNodeMenuPath | ( | QString const & | value | ) |
bool dnai::models::gui::Instruction::setOutputs | ( | const QList< models::gui::Output * > & | outputs | ) |
bool dnai::models::gui::Instruction::setUid | ( | quint32 | id | ) |
bool dnai::models::gui::Instruction::setX | ( | qint32 | x | ) |
bool dnai::models::gui::Instruction::setY | ( | qint32 | y | ) |
quint32 dnai::models::gui::Instruction::Uid | ( | ) | const |
qint32 dnai::models::gui::Instruction::x | ( | ) | const |
qint32 dnai::models::gui::Instruction::y | ( | ) | const |
Member Data Documentation
|
private |
Property Documentation
|
read |
|
readwrite |
|
readwrite |
The documentation for this class was generated from the following files:
- Gui/app/include/dnai/models/gui/instruction.h
- Gui/app/src/dnai/models/gui/instruction.cpp