Classes |
Signals |
Public Member Functions |
Protected Member Functions |
Properties |
Private Attributes |
List of all members
dnai::models::gui::declarable::Function Class Reference
#include <function.h>
Inheritance diagram for dnai::models::gui::declarable::Function:
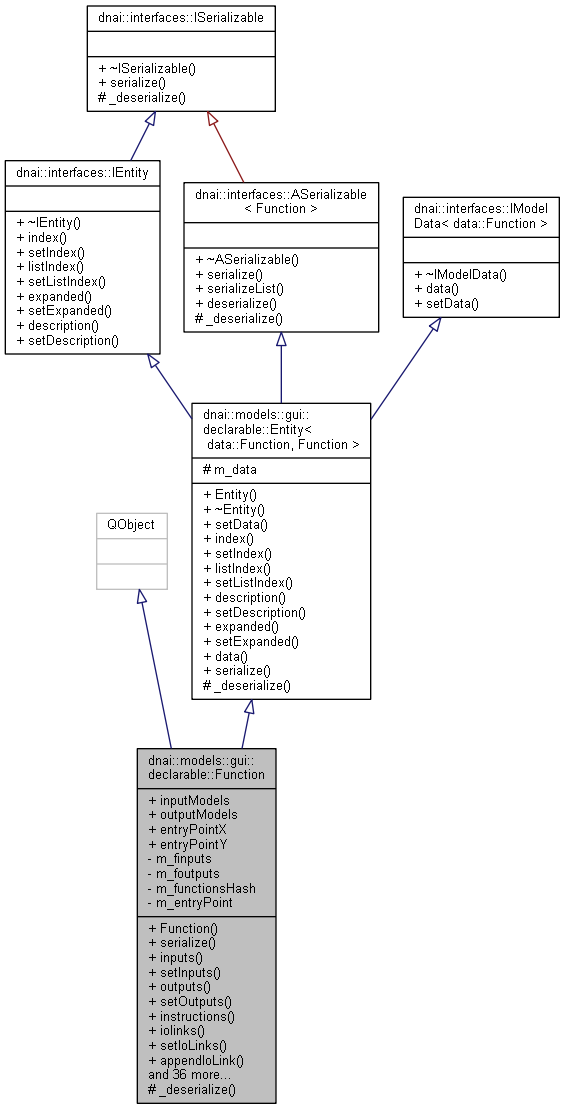
Collaboration diagram for dnai::models::gui::declarable::Function:
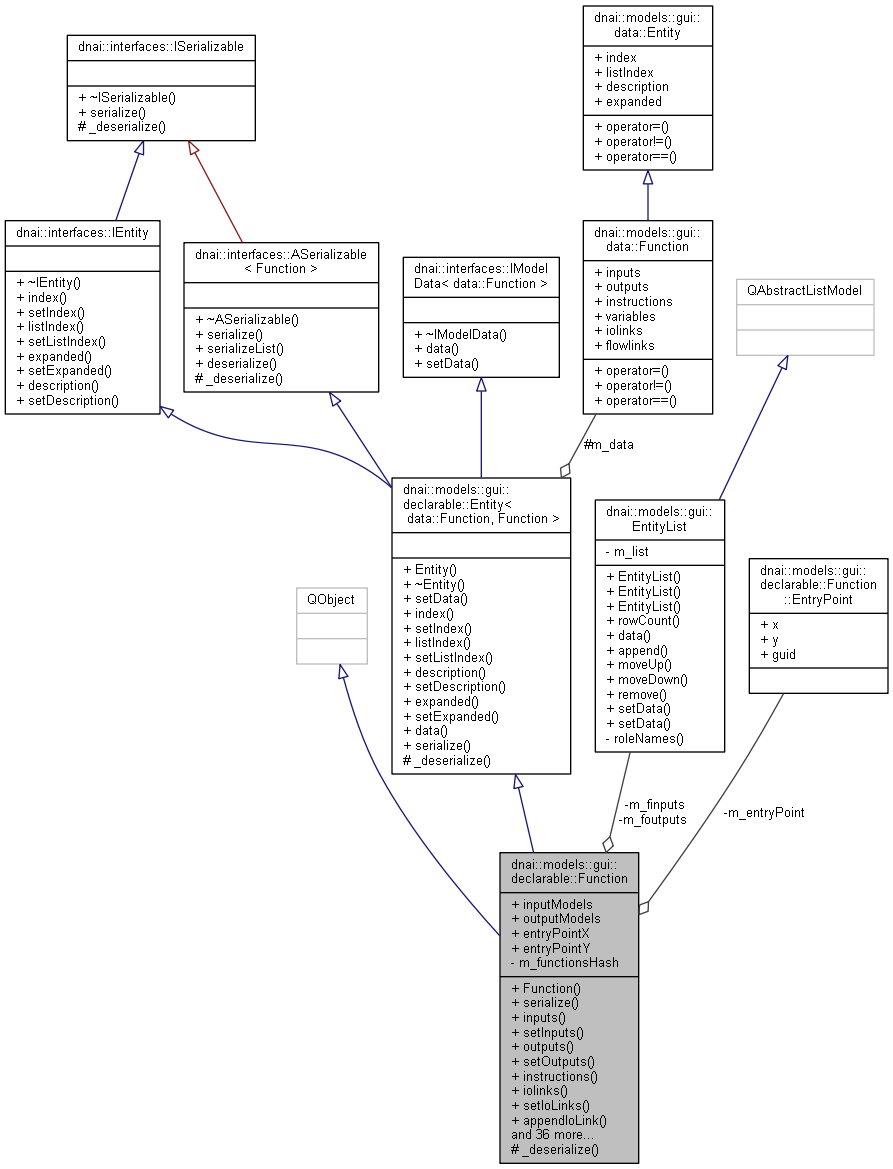
Classes | |
struct | EntryPoint |
Signals | |
void | inputModelsChanged (dnai::models::gui::EntityList *inputs) |
void | outputModelsChanged (dnai::models::gui::EntityList *outputs) |
Public Member Functions | |
Function (QObject *parent=nullptr) | |
void | serialize (QJsonObject &obj) const override |
Implement this function to serialize into QJsonObject. More... | |
const QList< models::Entity * > & | inputs () const |
bool | setInputs (const QList< models::Entity * > &inputs) |
const QList< models::Entity * > & | outputs () const |
bool | setOutputs (const QList< models::Entity * > &outputs) |
const QList< models::gui::Instruction * > & | instructions () const |
const QList< dnai::models::gui::IoLink * > & | iolinks () const |
bool | setIoLinks (const QList< dnai::models::gui::IoLink * > &) |
void | appendIoLink (dnai::models::gui::IoLink *) |
void | removeIoLink (dnai::models::gui::IoLink *link) |
Q_INVOKABLE dnai::models::gui::IoLink * | findIOLink (QUuid const &instruction, QString const &input) const |
const QList< dnai::models::gui::FlowLink * > & | flowlinks () const |
bool | setFlowLinks (const QList< dnai::models::gui::FlowLink * > &) |
void | appendFlowLink (dnai::models::gui::FlowLink *) |
void | removeFlowLink (dnai::models::gui::FlowLink *link) |
Q_INVOKABLE dnai::models::gui::FlowLink * | findFlowLink (QUuid const &from, quint32 outPin, QUuid const &to=QUuid()) const |
Q_INVOKABLE void | addInput (models::Entity *var) |
Q_INVOKABLE void | addOutput (models::Entity *var) |
Q_INVOKABLE void | removeInput (const QString &name) |
Q_INVOKABLE void | removeOutput (const QString &name) |
Q_INVOKABLE void | moveInputUp (int index) |
Q_INVOKABLE void | moveOutputUp (int index) |
Q_INVOKABLE void | moveInputDown (int index) |
Q_INVOKABLE void | moveOutputDown (int index) |
EntityList * | inputModels () const |
EntityList * | outputModels () const |
void | setInputModels (EntityList *inputs) |
void | setOutputModels (EntityList *outputs) |
void | addInstruction (Instruction *instruction) |
void | removeInstruction (Instruction *instruction) |
Instruction * | getInstruction (const QUuid &uuid) |
Q_INVOKABLE quint32 | getInputId (QString const &name) const |
Q_INVOKABLE quint32 | getOutputId (QString const &name) const |
models::Entity * | getInput (QString const &name) const |
models::Entity * | getOutput (QString const &name) const |
models::gui::Instruction * | getInstruction (quint32 uid) const |
models::gui::Instruction * | getInstruction (const QUuid &guid) const |
bool | hasInput (QString const &name, QUuid const &type=QUuid()) const |
bool | hasOutput (QString const &name, QUuid const &type=QUuid()) const |
models::gui::Instruction * | entryPoint () const |
void | setEntryPoint (QUuid uid) |
qint32 | entryPointX () const |
void | setEntryPointX (qint32 x) |
qint32 | entryPointY () const |
void | setEntryPointY (qint32 y) |
![]() | |
Entity ()=default | |
virtual | ~Entity () override=default |
virtual bool | setData (const data::Function &data) override |
set the data component of this object More... | |
virtual int | index () const override |
virtual bool | setIndex (const int index) override |
virtual QUuid | listIndex () const override |
virtual bool | setListIndex (QUuid listIndex) override |
virtual const QString & | description () const override |
virtual bool | setDescription (const QString &description) override |
virtual bool | expanded () const override |
virtual bool | setExpanded (bool exp) override |
virtual const data::Function & | data () const override |
Return the data component. More... | |
![]() | |
virtual | ~IEntity ()=default |
![]() | |
virtual | ~ISerializable ()=default |
![]() | |
virtual | ~IModelData ()=default |
virtual bool | setData (const data::Function &data)=0 |
set the data component of this object More... | |
![]() | |
virtual | ~ASerializable ()=default |
QJsonArray | serializeList (const QList< DataType * > &datalist) const |
Protected Member Functions | |
void | _deserialize (const QJsonObject &obj) override |
Implement this function in order to use deserialize(const QJsonObject &obj) More... | |
Properties | |
dnai::models::gui::EntityList | inputModels |
dnai::models::gui::EntityList | outputModels |
qint32 | entryPointX |
qint32 | entryPointY |
Private Attributes | |
EntityList * | m_finputs |
EntityList * | m_foutputs |
QHash< QUuid, Instruction * > | m_functionsHash |
EntryPoint | m_entryPoint |
Additional Inherited Members | |
![]() | |
static Function * | deserialize (const QJsonObject &obj, Args &...args) |
This function deserialize into a new instance of type T *. More... | |
![]() | |
data::Function | m_data |
Constructor & Destructor Documentation
|
explicit |
Member Function Documentation
|
overrideprotectedvirtual |
Implement this function in order to use deserialize(const QJsonObject &obj)
- Parameters
-
obj
Reimplemented from dnai::models::gui::declarable::Entity< data::Function, Function >.
void dnai::models::gui::declarable::Function::addInput | ( | models::Entity * | var | ) |
void dnai::models::gui::declarable::Function::addInstruction | ( | Instruction * | instruction | ) |
void dnai::models::gui::declarable::Function::addOutput | ( | models::Entity * | var | ) |
void dnai::models::gui::declarable::Function::appendFlowLink | ( | dnai::models::gui::FlowLink * | fl | ) |
void dnai::models::gui::declarable::Function::appendIoLink | ( | dnai::models::gui::IoLink * | link | ) |
Instruction * dnai::models::gui::declarable::Function::entryPoint | ( | ) | const |
qint32 dnai::models::gui::declarable::Function::entryPointX | ( | ) | const |
qint32 dnai::models::gui::declarable::Function::entryPointY | ( | ) | const |
FlowLink * dnai::models::gui::declarable::Function::findFlowLink | ( | QUuid const & | from, |
quint32 | outPin, | ||
QUuid const & | to = QUuid() |
||
) | const |
IoLink * dnai::models::gui::declarable::Function::findIOLink | ( | QUuid const & | instruction, |
QString const & | input | ||
) | const |
const QList< dnai::models::gui::FlowLink * > & dnai::models::gui::declarable::Function::flowlinks | ( | ) | const |
models::Entity * dnai::models::gui::declarable::Function::getInput | ( | QString const & | name | ) | const |
quint32 dnai::models::gui::declarable::Function::getInputId | ( | QString const & | name | ) | const |
Instruction * dnai::models::gui::declarable::Function::getInstruction | ( | const QUuid & | uuid | ) |
Instruction * dnai::models::gui::declarable::Function::getInstruction | ( | quint32 | uid | ) | const |
Instruction * dnai::models::gui::declarable::Function::getInstruction | ( | const QUuid & | guid | ) | const |
models::Entity * dnai::models::gui::declarable::Function::getOutput | ( | QString const & | name | ) | const |
quint32 dnai::models::gui::declarable::Function::getOutputId | ( | QString const & | name | ) | const |
bool dnai::models::gui::declarable::Function::hasInput | ( | QString const & | name, |
QUuid const & | type = QUuid() |
||
) | const |
bool dnai::models::gui::declarable::Function::hasOutput | ( | QString const & | name, |
QUuid const & | type = QUuid() |
||
) | const |
EntityList* dnai::models::gui::declarable::Function::inputModels | ( | ) | const |
|
signal |
const QList< models::Entity * > & dnai::models::gui::declarable::Function::inputs | ( | ) | const |
const QList< models::gui::Instruction * > & dnai::models::gui::declarable::Function::instructions | ( | ) | const |
const QList< dnai::models::gui::IoLink * > & dnai::models::gui::declarable::Function::iolinks | ( | ) | const |
void dnai::models::gui::declarable::Function::moveInputDown | ( | int | index | ) |
void dnai::models::gui::declarable::Function::moveInputUp | ( | int | index | ) |
void dnai::models::gui::declarable::Function::moveOutputDown | ( | int | index | ) |
void dnai::models::gui::declarable::Function::moveOutputUp | ( | int | index | ) |
EntityList* dnai::models::gui::declarable::Function::outputModels | ( | ) | const |
|
signal |
const QList< models::Entity * > & dnai::models::gui::declarable::Function::outputs | ( | ) | const |
void dnai::models::gui::declarable::Function::removeFlowLink | ( | dnai::models::gui::FlowLink * | link | ) |
void dnai::models::gui::declarable::Function::removeInput | ( | const QString & | name | ) |
void dnai::models::gui::declarable::Function::removeInstruction | ( | Instruction * | instruction | ) |
void dnai::models::gui::declarable::Function::removeIoLink | ( | dnai::models::gui::IoLink * | link | ) |
void dnai::models::gui::declarable::Function::removeOutput | ( | const QString & | name | ) |
|
overridevirtual |
Implement this function to serialize into QJsonObject.
- Parameters
-
obj
Reimplemented from dnai::models::gui::declarable::Entity< data::Function, Function >.
void dnai::models::gui::declarable::Function::setEntryPoint | ( | QUuid | uid | ) |
void dnai::models::gui::declarable::Function::setEntryPointX | ( | qint32 | x | ) |
void dnai::models::gui::declarable::Function::setEntryPointY | ( | qint32 | y | ) |
bool dnai::models::gui::declarable::Function::setFlowLinks | ( | const QList< dnai::models::gui::FlowLink * > & | fl | ) |
void dnai::models::gui::declarable::Function::setInputModels | ( | EntityList * | inputs | ) |
bool dnai::models::gui::declarable::Function::setInputs | ( | const QList< models::Entity * > & | inputs | ) |
bool dnai::models::gui::declarable::Function::setIoLinks | ( | const QList< dnai::models::gui::IoLink * > & | value | ) |
void dnai::models::gui::declarable::Function::setOutputModels | ( | EntityList * | outputs | ) |
bool dnai::models::gui::declarable::Function::setOutputs | ( | const QList< models::Entity * > & | outputs | ) |
Member Data Documentation
|
private |
|
private |
|
private |
|
private |
Property Documentation
|
readwrite |
|
readwrite |
|
readwrite |
|
readwrite |
The documentation for this class was generated from the following files:
- Gui/app/include/dnai/models/gui/declarable/function.h
- Gui/app/GeneratedFiles/Debug/moc_function.cpp
- Gui/app/src/dnai/models/gui/declarable/function.cpp