Public Slots |
Signals |
Public Member Functions |
Static Public Member Functions |
Protected Member Functions |
Properties |
Private Attributes |
Static Private Attributes |
List of all members
dnai::Editor Class Reference
#include <editor.h>
Inheritance diagram for dnai::Editor:
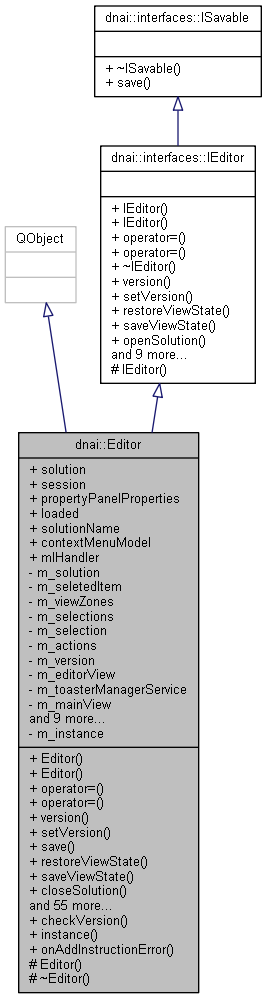
Collaboration diagram for dnai::Editor:
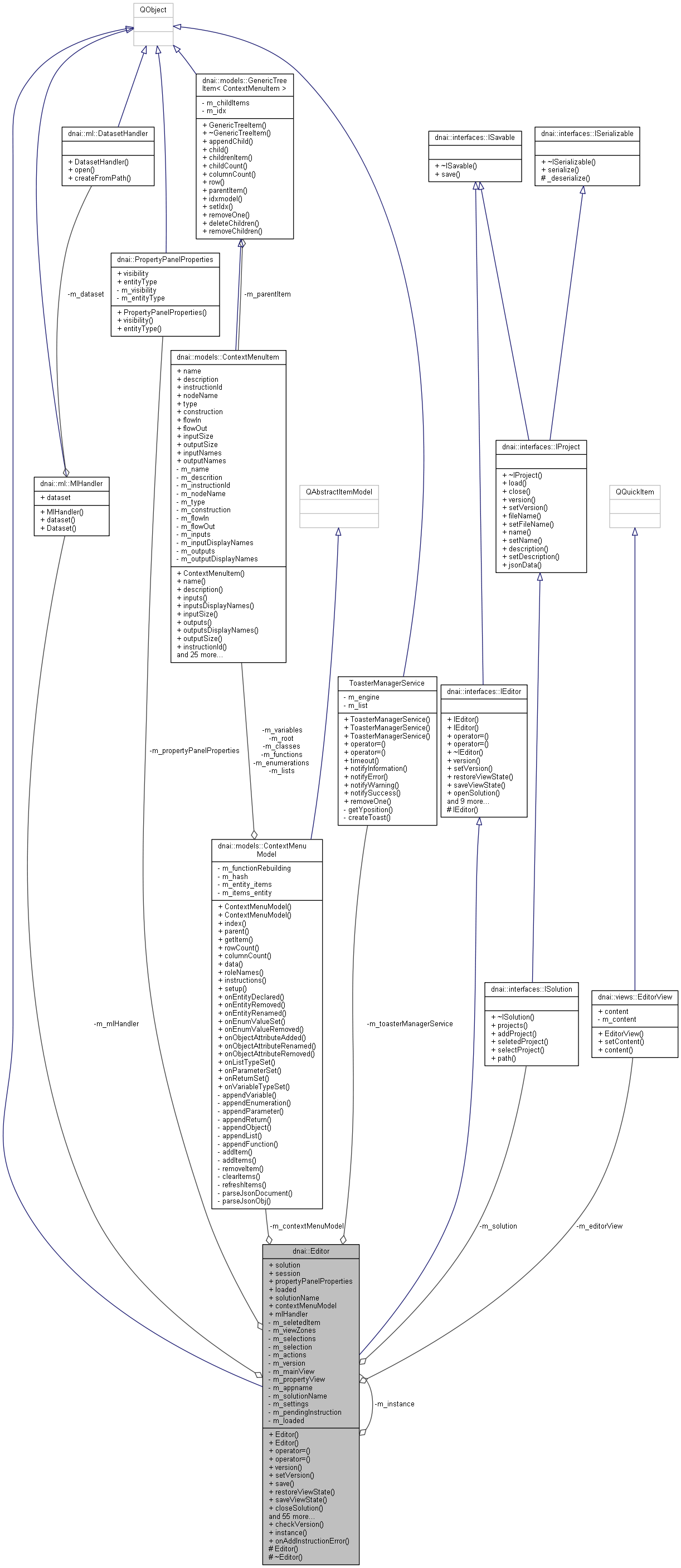
Public Slots | |
void | onAddInstructionError (quint32 func, quint32 type, QList< quint32 > const &args, QString const &msg) |
Signals | |
void | solutionChanged (dnai::Solution *proj) |
void | loadedChanged (bool) |
void | contextMenuModelChanged (dnai::models::ContextMenuModel *m) const |
Public Member Functions | |
Editor (Editor const &)=delete | |
Editor (Editor &&)=delete | |
Editor & | operator= (Editor const &)=delete |
Editor & | operator= (Editor &&)=delete |
const QString & | version () const override |
void | setVersion (const QString &version) override |
void | save () override |
void | restoreViewState (const QJsonObject &obj) override |
void | saveViewState () override |
void | closeSolution () override |
const QList< interfaces::ICommand * > & | actions () const override |
const QObject & | selection () const override |
const QList< QObject * > & | selections () const override |
interfaces::ISolution * | solution () const override |
dnai::Solution * | getSolution () const |
void | addView (QQuickItem *v) override |
views::EditorView * | mainView () const |
bool | loaded () const |
QString const & | solutionName () const |
void | loadContextMenuModel () |
dnai::models::ContextMenuModel * | contextMenuModel () const |
void | setContextMenuModel (dnai::models::ContextMenuModel *ctx) |
Q_INVOKABLE void | updateContextMenuModel (dnai::models::Entity *entity) const |
void | setLoaded (bool) |
Q_INVOKABLE void | registerEditorView (views::EditorView *view) |
Q_INVOKABLE const QList< QQuickItem * > & | views () const override |
Q_INVOKABLE QQuickItem * | selectedView () const override |
Q_INVOKABLE bool | isSolutionLoad () const |
Q_INVOKABLE void | selectView (QQuickItem *i) |
Q_INVOKABLE void | createNode (dnai::models::Entity *entity, dnai::models::ContextMenuItem *node, qint32 x, qint32 y) |
Q_INVOKABLE void | openSolution () override |
Q_INVOKABLE void | loadSolution (const QString &filename) override |
Q_INVOKABLE void | newEditor (const QString &solutionToLoad="") |
Q_INVOKABLE void | notifyInformation (QString const &, std::function< void()> func=[](){}) |
Q_INVOKABLE void | notifySuccess (QString const &text, std::function< void()> func=[](){}) |
Q_INVOKABLE void | notifyError (QString const &text, std::function< void()> func=[](){}) |
Q_INVOKABLE void | notifyWarning (QString const &text, std::function< void()> func=[](){}) |
Q_INVOKABLE void | buildSolution () |
Q_INVOKABLE void | startApp () |
Q_INVOKABLE void | registerMainView (QObject *) |
Q_INVOKABLE void | addProject (QString const &proj_name, QString const &proj_desc) |
Q_INVOKABLE bool | createSolution (const QString &name, const QString &description, const QString &path, const QString &proj_name, const QString &proj_desc) |
Q_INVOKABLE QQuickWindow * | mainView () |
Q_INVOKABLE QQuickItem * | qmlMainView () |
Q_INVOKABLE void | registerPropertyView (QQuickItem *view) |
Q_INVOKABLE QQuickItem * | propertyView () const |
Q_INVOKABLE void | loadFunction (dnai::models::Entity *entity) const |
Q_INVOKABLE QSettings * | settings () |
Q_INVOKABLE void | registerSettings (QSettings *settings) |
Q_INVOKABLE bool | isNewVersionAvailable () const |
Q_INVOKABLE qreal | getSettingNumber (const QString &path) |
void | selectProject (Project *proj) |
PropertyPanelProperties * | propertyPanelProperties () |
void | setSolution (dnai::Solution *sol) |
Session * | session () const |
void | setAppName (QString const &name) |
void | setSolutionName (QString const &name) |
dnai::ml::MlHandler * | mlHandler () |
Q_INVOKABLE QQuickItem * | createNodeQMLComponent (dnai::models::Entity *func, dnai::models::gui::Instruction *instruction, QQuickItem *parent) const |
Q_INVOKABLE void | setAsEntryPoint (dnai::views::GenericNode *instruction, dnai::views::GenericNode *entry) |
Q_INVOKABLE void | createFlowLink (dnai::views::GenericNode *from, dnai::views::GenericNode *to, dnai::models::Entity *func, dnai::models::gui::Instruction *fromIns, qint32 outpin, dnai::models::gui::Instruction *toIns) const |
Q_INVOKABLE void | removeFlowLink (dnai::views::GenericNode *instruction, qint32 outpin) const |
Q_INVOKABLE void | createIOLink (dnai::views::GenericNode *from, dnai::views::GenericNode *to, dnai::models::Entity *func, dnai::models::gui::Instruction *instr, QString input) const |
Q_INVOKABLE void | removeIOLink (dnai::views::GenericNode *instruction, dnai::models::gui::Instruction *instr, QString input) const |
Q_INVOKABLE void | finishInstructionBuilding (dnai::models::Entity *func, dnai::models::gui::Instruction *instr) |
![]() | |
IEditor (IEditor const &)=delete | |
IEditor (IEditor &&)=delete | |
IEditor & | operator= (IEditor const &)=delete |
IEditor & | operator= (IEditor &&)=delete |
virtual | ~IEditor ()=default |
![]() | |
virtual | ~ISavable ()=default |
Static Public Member Functions | |
static Q_INVOKABLE void | checkVersion () |
static Editor & | instance () |
Protected Member Functions | |
Editor () | |
~Editor () | |
![]() | |
IEditor ()=default | |
Private Attributes | |
interfaces::ISolution * | m_solution |
QQuickItem * | m_seletedItem = nullptr |
QList< QQuickItem * > | m_viewZones |
QList< QObject * > | m_selections |
QObject * | m_selection |
QList< interfaces::ICommand * > | m_actions |
QString | m_version = "0.0.1" |
views::EditorView * | m_editorView |
ToasterManagerService | m_toasterManagerService |
QQuickWindow * | m_mainView = nullptr |
QQuickItem * | m_propertyView |
dnai::PropertyPanelProperties * | m_propertyPanelProperties |
QString | m_appname |
QString | m_solutionName |
dnai::models::ContextMenuModel * | m_contextMenuModel |
QSettings * | m_settings |
dnai::ml::MlHandler | m_mlHandler |
std::queue< std::tuple< models::ContextMenuItem *, quint32, quint32 > > | m_pendingInstruction |
bool | m_loaded = false |
Static Private Attributes | |
static Editor * | m_instance = nullptr |
Constructor & Destructor Documentation
|
protected |
|
protected |
|
delete |
|
delete |
Member Function Documentation
|
overridevirtual |
Implements dnai::interfaces::IEditor.
void dnai::Editor::addProject | ( | QString const & | proj_name, |
QString const & | proj_desc | ||
) |
|
overridevirtual |
Implements dnai::interfaces::IEditor.
void dnai::Editor::buildSolution | ( | ) |
|
static |
|
overridevirtual |
Implements dnai::interfaces::IEditor.
dnai::models::ContextMenuModel* dnai::Editor::contextMenuModel | ( | ) | const |
|
signal |
void dnai::Editor::createFlowLink | ( | dnai::views::GenericNode * | from, |
dnai::views::GenericNode * | to, | ||
dnai::models::Entity * | func, | ||
dnai::models::gui::Instruction * | fromIns, | ||
qint32 | outpin, | ||
dnai::models::gui::Instruction * | toIns | ||
) | const |
void dnai::Editor::createIOLink | ( | dnai::views::GenericNode * | from, |
dnai::views::GenericNode * | to, | ||
dnai::models::Entity * | func, | ||
dnai::models::gui::Instruction * | instr, | ||
QString | input | ||
) | const |
void dnai::Editor::createNode | ( | dnai::models::Entity * | entity, |
dnai::models::ContextMenuItem * | node, | ||
qint32 | x, | ||
qint32 | y | ||
) |
QQuickItem * dnai::Editor::createNodeQMLComponent | ( | dnai::models::Entity * | func, |
dnai::models::gui::Instruction * | instruction, | ||
QQuickItem * | parent | ||
) | const |
bool dnai::Editor::createSolution | ( | const QString & | name, |
const QString & | description, | ||
const QString & | path, | ||
const QString & | proj_name, | ||
const QString & | proj_desc | ||
) |
void dnai::Editor::finishInstructionBuilding | ( | dnai::models::Entity * | func, |
dnai::models::gui::Instruction * | instr | ||
) |
qreal dnai::Editor::getSettingNumber | ( | const QString & | path | ) |
Solution * dnai::Editor::getSolution | ( | ) | const |
|
static |
bool dnai::Editor::isNewVersionAvailable | ( | ) | const |
bool dnai::Editor::isSolutionLoad | ( | ) | const |
void dnai::Editor::loadContextMenuModel | ( | ) |
bool dnai::Editor::loaded | ( | ) | const |
|
signal |
void dnai::Editor::loadFunction | ( | dnai::models::Entity * | entity | ) | const |
|
overridevirtual |
Implements dnai::interfaces::IEditor.
views::EditorView * dnai::Editor::mainView | ( | ) | const |
QQuickWindow * dnai::Editor::mainView | ( | ) |
dnai::ml::MlHandler* dnai::Editor::mlHandler | ( | ) |
void dnai::Editor::newEditor | ( | const QString & | solutionToLoad = "" | ) |
void dnai::Editor::notifyError | ( | QString const & | text, |
std::function< void()> | func = []() {} |
||
) |
void dnai::Editor::notifyInformation | ( | QString const & | text, |
std::function< void()> | func = [](){} |
||
) |
void dnai::Editor::notifySuccess | ( | QString const & | text, |
std::function< void()> | func = []() {} |
||
) |
void dnai::Editor::notifyWarning | ( | QString const & | text, |
std::function< void()> | func = []() {} |
||
) |
|
slot |
|
overridevirtual |
Implements dnai::interfaces::IEditor.
PropertyPanelProperties* dnai::Editor::propertyPanelProperties | ( | ) |
QQuickItem * dnai::Editor::propertyView | ( | ) | const |
QQuickItem * dnai::Editor::qmlMainView | ( | ) |
void dnai::Editor::registerEditorView | ( | views::EditorView * | view | ) |
void dnai::Editor::registerMainView | ( | QObject * | mainView | ) |
void dnai::Editor::registerPropertyView | ( | QQuickItem * | view | ) |
void dnai::Editor::registerSettings | ( | QSettings * | settings | ) |
void dnai::Editor::removeFlowLink | ( | dnai::views::GenericNode * | instruction, |
qint32 | outpin | ||
) | const |
void dnai::Editor::removeIOLink | ( | dnai::views::GenericNode * | instruction, |
dnai::models::gui::Instruction * | instr, | ||
QString | input | ||
) | const |
|
overridevirtual |
Implements dnai::interfaces::IEditor.
|
overridevirtual |
Implements dnai::interfaces::ISavable.
|
overridevirtual |
Implements dnai::interfaces::IEditor.
|
overridevirtual |
Implements dnai::interfaces::IEditor.
|
overridevirtual |
Implements dnai::interfaces::IEditor.
|
overridevirtual |
Implements dnai::interfaces::IEditor.
void dnai::Editor::selectProject | ( | Project * | proj | ) |
void dnai::Editor::selectView | ( | QQuickItem * | i | ) |
Session* dnai::Editor::session | ( | ) | const |
void dnai::Editor::setAppName | ( | QString const & | name | ) |
void dnai::Editor::setAsEntryPoint | ( | dnai::views::GenericNode * | instruction, |
dnai::views::GenericNode * | entry | ||
) |
void dnai::Editor::setContextMenuModel | ( | dnai::models::ContextMenuModel * | ctx | ) |
void dnai::Editor::setLoaded | ( | bool | newLoaded | ) |
void dnai::Editor::setSolution | ( | dnai::Solution * | sol | ) |
void dnai::Editor::setSolutionName | ( | QString const & | name | ) |
QSettings * dnai::Editor::settings | ( | ) |
|
overridevirtual |
Implements dnai::interfaces::IEditor.
|
overridevirtual |
Implements dnai::interfaces::IEditor.
|
signal |
QString const& dnai::Editor::solutionName | ( | ) | const |
void dnai::Editor::startApp | ( | ) |
void dnai::Editor::updateContextMenuModel | ( | dnai::models::Entity * | entity | ) | const |
|
overridevirtual |
Implements dnai::interfaces::IEditor.
|
overridevirtual |
Implements dnai::interfaces::IEditor.
Member Data Documentation
|
private |
|
private |
|
private |
|
private |
|
staticprivate |
|
private |
|
private |
|
private |
|
private |
|
private |
|
private |
|
private |
|
private |
|
private |
|
private |
|
private |
|
private |
|
private |
|
private |
|
private |
Property Documentation
|
readwrite |
|
readwrite |
|
read |
|
read |
|
read |
|
readwrite |
|
read |
The documentation for this class was generated from the following files:
- Gui/app/include/dnai/editor.h
- Gui/app/GeneratedFiles/Debug/moc_editor.cpp
- Gui/app/src/dnai/editor.cpp