Public Slots |
Signals |
Public Member Functions |
Static Public Member Functions |
Private Member Functions |
Private Attributes |
List of all members
dnai::gcore::InstructionHandler Class Reference
#include <instructionhandler.h>
Inheritance diagram for dnai::gcore::InstructionHandler:
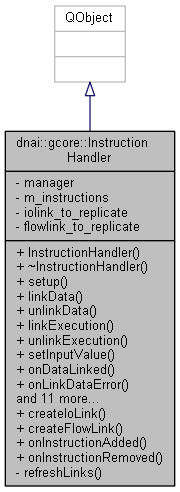
Collaboration diagram for dnai::gcore::InstructionHandler:
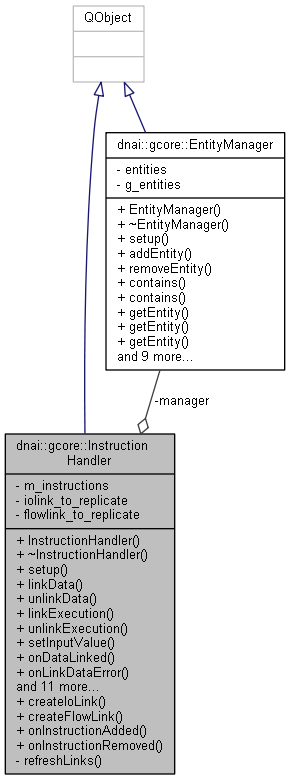
Public Slots | |
void | onInstructionAdded (dnai::models::Entity *func, dnai::models::gui::Instruction *instruction) |
void | onInstructionRemoved (dnai::models::Entity *func, dnai::models::gui::Instruction *instruction) |
Signals | |
void | dataLinked (dnai::models::Entity *func, dnai::models::gui::Instruction *from, QString output, dnai::models::gui::Instruction *to, QString input) |
void | dataUnlinked (dnai::models::Entity *func, dnai::models::gui::Instruction *instruction, QString input) |
void | executionLinked (dnai::models::Entity *func, dnai::models::gui::Instruction *from, quint32 outPin, dnai::models::gui::Instruction *to) |
void | executionUnlinked (dnai::models::Entity *func, dnai::models::gui::Instruction *from, quint32 outPin) |
void | inputValueSet (dnai::models::Entity *func, dnai::models::gui::Instruction *instruction, QString input, QString value) |
Public Member Functions | |
InstructionHandler (EntityManager &manager) | |
~InstructionHandler ()=default | |
void | setup () |
Q_INVOKABLE void | linkData (quint32 function, quint32 instruction, QString const &input, quint32 fromInstruction, QString const &output, bool save=true) |
Q_INVOKABLE void | unlinkData (quint32 function, quint32 instruction, QString const &input, bool save=true) |
Q_INVOKABLE void | linkExecution (quint32 function, quint32 instruction, quint32 outPin, quint32 toInstruction, bool save=true) |
Q_INVOKABLE void | unlinkExecution (quint32 function, quint32 instruction, quint32 outPin, bool save=true) |
Q_INVOKABLE void | setInputValue (quint32 function, quint32 instruction, QString const &input, QString const &value, bool save=true) |
void | onDataLinked (quint32 function, quint32 from, QString const &output, quint32 to, QString const &input) |
void | onLinkDataError (quint32 function, quint32 from, QString const &output, quint32 to, QString const &input, QString const &message) |
void | onDataUnlinked (quint32 function, quint32 instruction, QString const &input) |
void | onUnlinkDataError (quint32 function, quint32 instruction, QString const &input, QString const &message) |
void | onExecutionLinked (quint32 function, quint32 instruction, quint32 outpin, quint32 toInstruction) |
void | onLinkExecutionError (quint32 function, quint32 instruction, quint32 outpin, quint32 toInstruction, QString const &message) |
void | onExecutionUnlinked (quint32 function, quint32 instruction, quint32 outpin) |
void | onUnlinkExecutionError (quint32 function, quint32 instruction, quint32 outpin, QString const &message) |
void | onInputValueSet (quint32 function, quint32 instruction, QString const &input, QString const &value) |
void | onSetInputValueError (quint32 function, quint32 instruction, QString const &input, QString const &value, QString const &msg) |
bool | contains (QUuid const &instGuid) const |
models::gui::Instruction * | getInstruction (QUuid const &guid) const |
QList< models::gui::Instruction * > | getInstructionsOfPath (QString const &nodeMenupath) const |
Static Public Member Functions | |
static models::gui::IoLink * | createIoLink (QUuid const &from, QString const &output, QUuid const &to, QString const &input) |
static models::gui::FlowLink * | createFlowLink (QUuid const &from, int outindex, QUuid const &to) |
Private Member Functions | |
void | refreshLinks () |
Private Attributes | |
EntityManager & | manager |
QHash< QUuid, models::gui::Instruction * > | m_instructions |
std::unordered_map< models::gui::IoLink *, models::Entity * > | iolink_to_replicate |
std::unordered_map< models::gui::FlowLink *, models::Entity * > | flowlink_to_replicate |
Constructor & Destructor Documentation
dnai::gcore::InstructionHandler::InstructionHandler | ( | EntityManager & | manager | ) |
|
default |
Member Function Documentation
bool dnai::gcore::InstructionHandler::contains | ( | QUuid const & | instGuid | ) | const |
|
static |
|
static |
|
signal |
|
signal |
|
signal |
|
signal |
models::gui::Instruction * dnai::gcore::InstructionHandler::getInstruction | ( | QUuid const & | guid | ) | const |
QList< models::gui::Instruction * > dnai::gcore::InstructionHandler::getInstructionsOfPath | ( | QString const & | nodeMenupath | ) | const |
|
signal |
void dnai::gcore::InstructionHandler::linkData | ( | quint32 | function, |
quint32 | instruction, | ||
QString const & | input, | ||
quint32 | fromInstruction, | ||
QString const & | output, | ||
bool | save = true |
||
) |
void dnai::gcore::InstructionHandler::linkExecution | ( | quint32 | function, |
quint32 | instruction, | ||
quint32 | outPin, | ||
quint32 | toInstruction, | ||
bool | save = true |
||
) |
void dnai::gcore::InstructionHandler::onDataLinked | ( | quint32 | function, |
quint32 | from, | ||
QString const & | output, | ||
quint32 | to, | ||
QString const & | input | ||
) |
void dnai::gcore::InstructionHandler::onDataUnlinked | ( | quint32 | function, |
quint32 | instruction, | ||
QString const & | input | ||
) |
void dnai::gcore::InstructionHandler::onExecutionLinked | ( | quint32 | function, |
quint32 | instruction, | ||
quint32 | outpin, | ||
quint32 | toInstruction | ||
) |
void dnai::gcore::InstructionHandler::onExecutionUnlinked | ( | quint32 | function, |
quint32 | instruction, | ||
quint32 | outpin | ||
) |
void dnai::gcore::InstructionHandler::onInputValueSet | ( | quint32 | function, |
quint32 | instruction, | ||
QString const & | input, | ||
QString const & | value | ||
) |
|
slot |
|
slot |
void dnai::gcore::InstructionHandler::onLinkDataError | ( | quint32 | function, |
quint32 | from, | ||
QString const & | output, | ||
quint32 | to, | ||
QString const & | input, | ||
QString const & | message | ||
) |
void dnai::gcore::InstructionHandler::onLinkExecutionError | ( | quint32 | function, |
quint32 | instruction, | ||
quint32 | outpin, | ||
quint32 | toInstruction, | ||
QString const & | message | ||
) |
void dnai::gcore::InstructionHandler::onSetInputValueError | ( | quint32 | function, |
quint32 | instruction, | ||
QString const & | input, | ||
QString const & | value, | ||
QString const & | msg | ||
) |
void dnai::gcore::InstructionHandler::onUnlinkDataError | ( | quint32 | function, |
quint32 | instruction, | ||
QString const & | input, | ||
QString const & | message | ||
) |
void dnai::gcore::InstructionHandler::onUnlinkExecutionError | ( | quint32 | function, |
quint32 | instruction, | ||
quint32 | outpin, | ||
QString const & | message | ||
) |
|
private |
void dnai::gcore::InstructionHandler::setInputValue | ( | quint32 | function, |
quint32 | instruction, | ||
QString const & | input, | ||
QString const & | value, | ||
bool | save = true |
||
) |
void dnai::gcore::InstructionHandler::setup | ( | ) |
void dnai::gcore::InstructionHandler::unlinkData | ( | quint32 | function, |
quint32 | instruction, | ||
QString const & | input, | ||
bool | save = true |
||
) |
void dnai::gcore::InstructionHandler::unlinkExecution | ( | quint32 | function, |
quint32 | instruction, | ||
quint32 | outPin, | ||
bool | save = true |
||
) |
Member Data Documentation
|
private |
|
private |
|
private |
|
private |
The documentation for this class was generated from the following files:
- Gui/app/include/dnai/core/instructionhandler.h
- Gui/app/GeneratedFiles/Debug/moc_instructionhandler.cpp
- Gui/app/src/dnai/core/instructionhandler.cpp